Overview & Getting Started
Welcome to Haxelo SDK documentation, this will guide you to quickly integrate and utilize the SDK functionalities within your games.
Basics
Lets get started
Registration
First of all we need to add your first game on our platform
Download SDK
Here you can download relevant version of Haxelo SDK
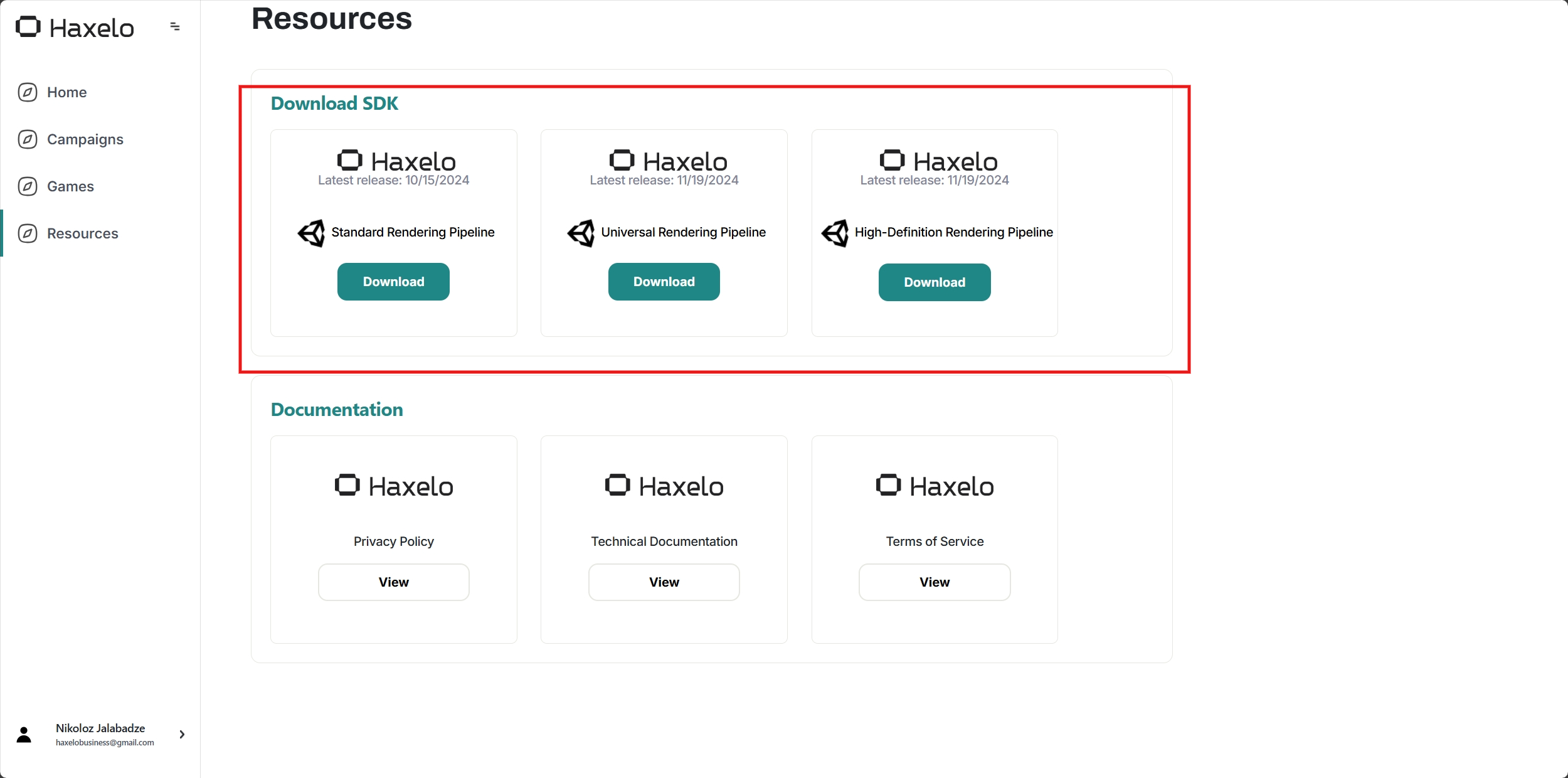
Import it into your Unity project.
Insert keys into Haxelo editor
You can copy your api key and Identifier anytime from here
Insert keys into the editor.
To open Haxelo editor, from the main menu bar find Haxelo, and click on Configure.
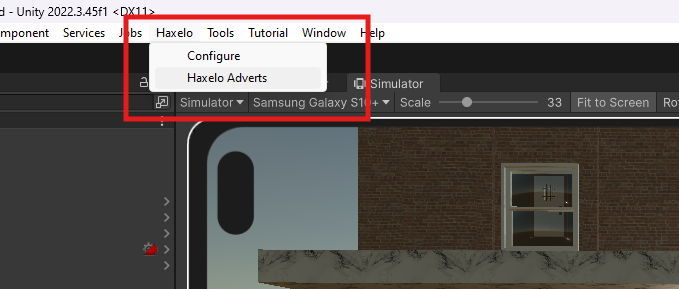
Enter your API key and Game ID, then click apply and wait for the verification process. If it's succeeded, you should see the message “API Key applied successfully!”.
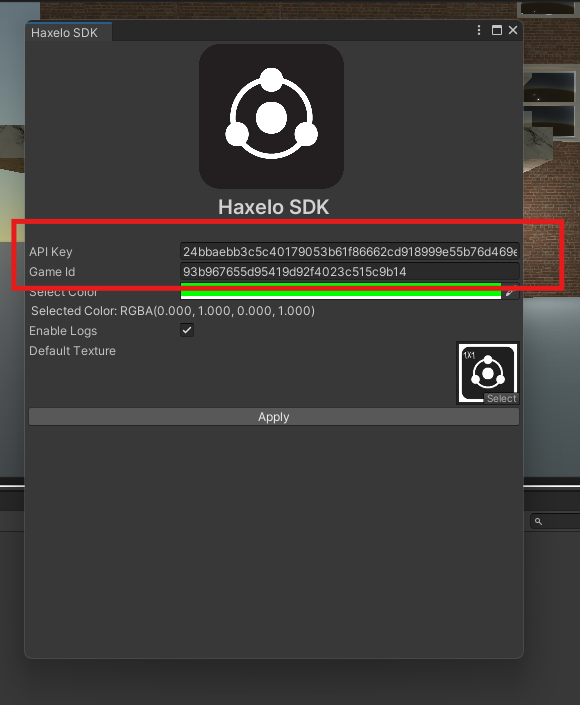
Haxelo Configuration
You can open Haxelo configuration window by navigating to Haxelo tab at the top.
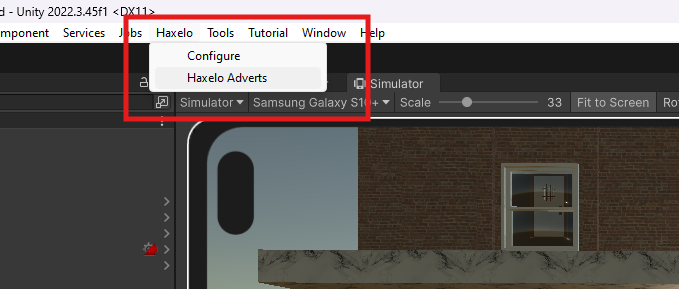
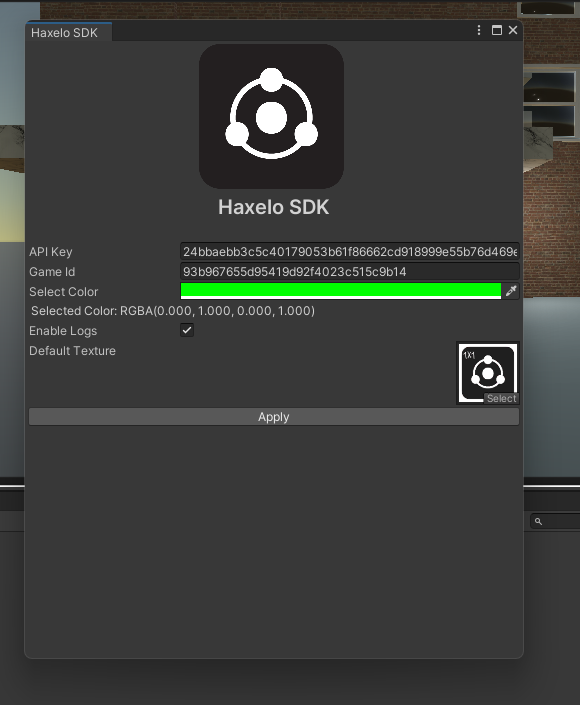
After setup is done, the configuration has several tools that you can configure.
Outline Color
You can select your default global outline color from here, however, you can override this setting from the banner configuration. Outlines only appear on banners that are clickable and have widgets.
Logs
You can enable/disable haxelo logs from this configuration.
Default Texture
You can select default texture for banners, if there are no ads, this will be shown.
Placing billboards
To get started with banners, from haxelo menu open Haxelo Adverts.
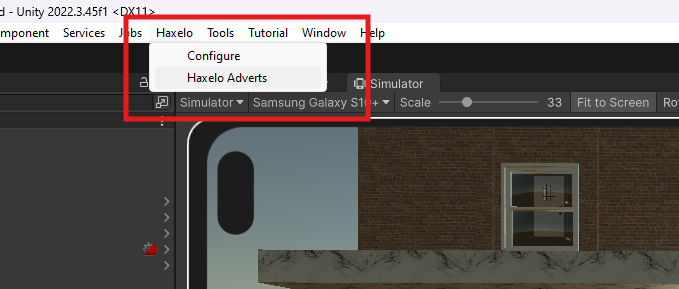
This window should pop up.
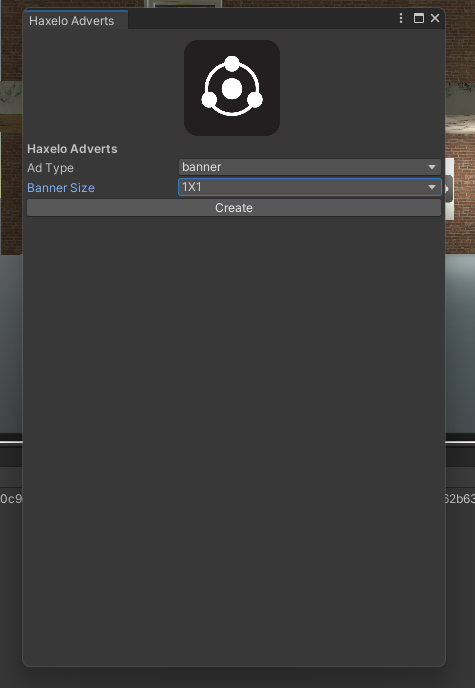
Select your Ad Type and banner size. Then click on Create. The banner should appear in your scene. Position it as you would like, but keep in mind that you can't modify the aspect ratio of the banner.
Banner Configuration
Each banner can be separately configured, but this is not a required step. They all have their default values, if required, you can configure each of them.
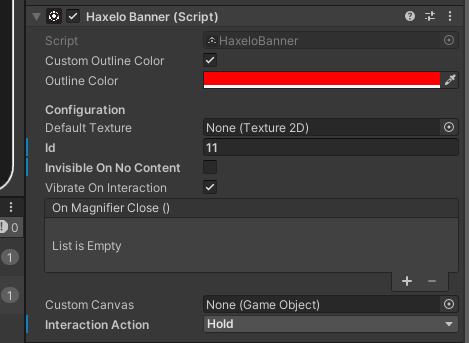
By enabling custom outline color, you can override the global color value.
Default texture can also be overridden from banner configuration.
PLEASE DO NOT MODIFY ID, THIS IS FOR BANNER IDENTIFICATION AND CHANGING IT WILL CAUSE PROBLEMS.
Invisible on no content means that your banner will not be visible in the game if there is no ad to show. by default, it's disabled.
You are also able to turn on/off vibration on interaction for mobile devices only.
On magnifier close callback can be used to do any action when the user closes the advertisement of this banner.
Interaction type can also be configured from banner configration.
If needed, you can also set up your custom canvas for magnifier and assign it in the inspector of banner.
In your resources folder, you will find the default prefab. Duplicate it and redesign it for your needs. Please do not remove or modify the default prefab to avoid errors.
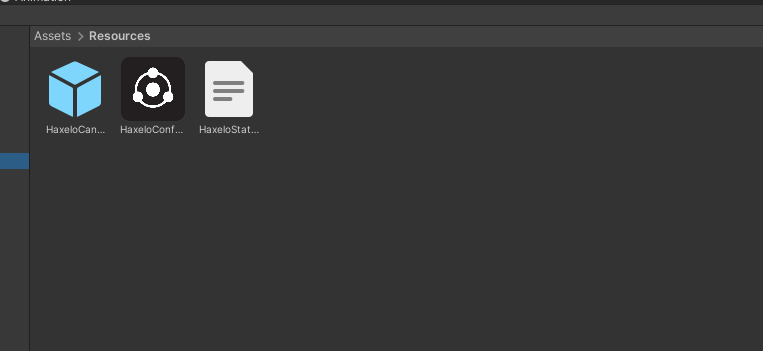
Also please keep the canvas structure and button functions the same, otherwise it will not function as expected.
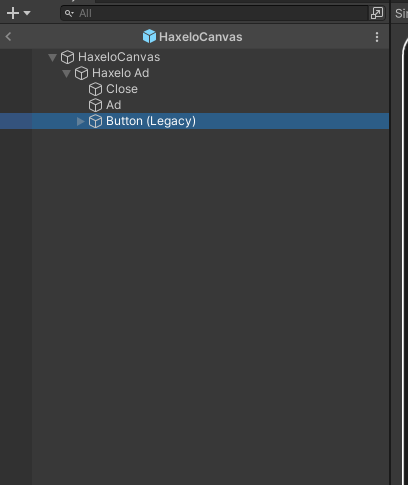
Haxelo SDK Methods
In the project, there is sample initialization script that will show you how you can initilize Haxelo Session and use all of the methods.